PRECODE ESR Bioinformatics Course
2021-02-20
Chapter 1 Prerequisites
This book provides an introductory course in R programming. It is primarily designed for wet lab biologists who are interested in developing core skills in the R programming language and who would like to analyze their own RNAseq data!
1.1 Installing R and RStudio
To complete this course you will need to install R and RStudio on your local machine. Detailed instructions on how to install R and RStudio are provided in “Hands-on programming with R”.
Hands-on programming with R is an excellent open source reference for learning the basics of R and can be used as a companion to this course. Please also find additional learning resources below that can be used as companions to this course.
We will use RStudio in this course. RStudio is an integrated development environment (IDE) for R. It includes a console, syntax-highlighting editor that supports direct code execution, as well as tools for plotting, history, debugging and workspace management.
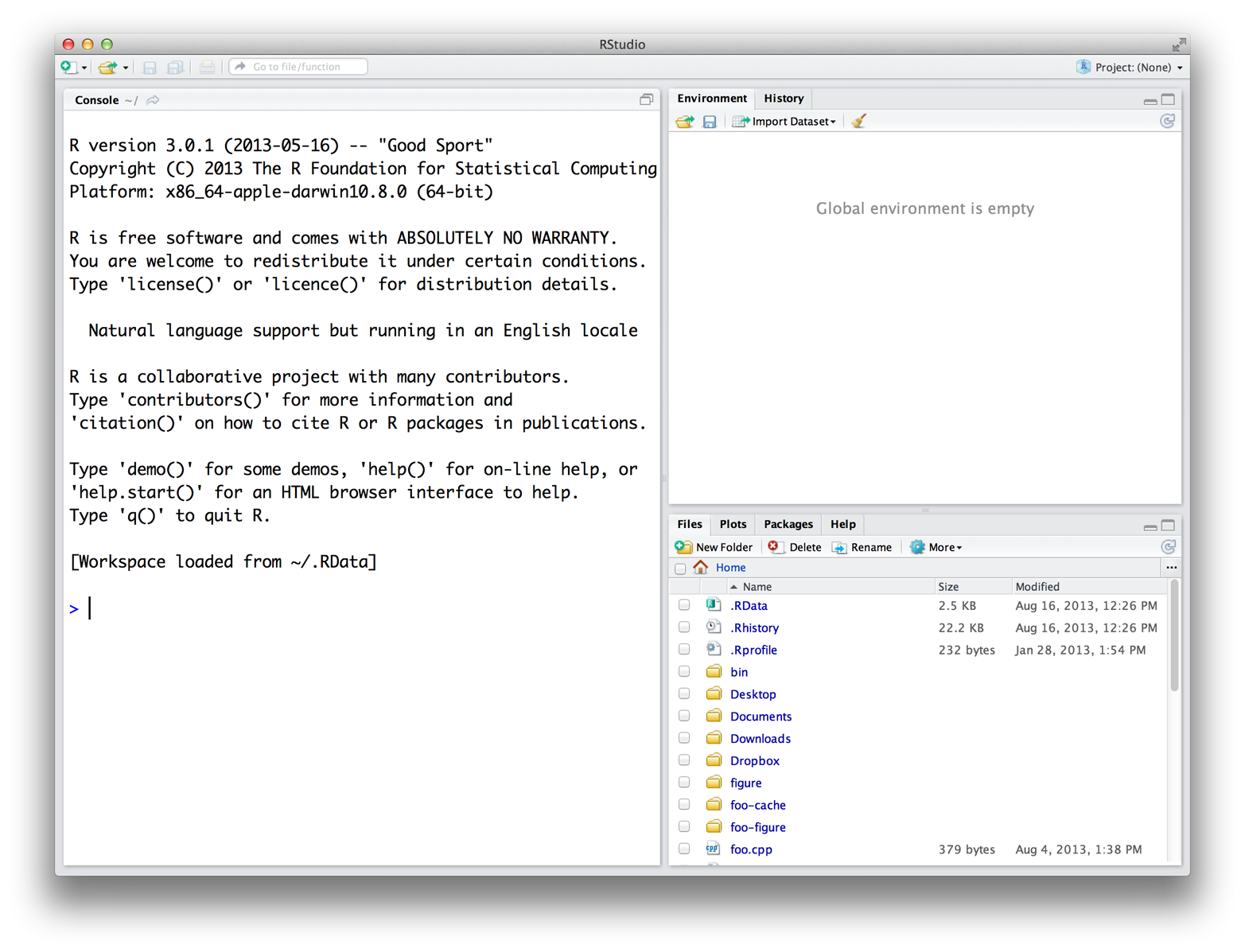
Figure 1.1: The RStudio IDE for R.
1.2 Installing R and Bioconductor packages
CRAN (the Comprehensive R Archive Network) is a repository of packages for R, and it is mirrored on many servers around the world. It is the default repository system used by R.
You can install a package from CRAN by using install.packages()
. To install ggplot2
use:
install.packages("ggplot2")
If you are prompted to select a download mirror use the first choice, https://cloud.r-project.org/.
You can also install multiple packages at once by passing the function a vector of package names:
install.packages(c("ggplot2", "tidyverse"))
Once you have R/RStudio up and running please also install Bioconductor. Bioconductor is a repository of R packages for analyzing genomic data!
Bioconductor can be installed easily using the following script:
if (!requireNamespace("BiocManager", quietly = TRUE))
install.packages("BiocManager")
::install(version = "3.12") BiocManager
Individual packages of interest can then be installed as follows:
::install("DESeq2") BiocManager
Multiple packages of interest can be installed as follows:
::install(c("DESeq2", "ggpubr", "ComplexHeatmap",
BiocManager"circlize", "clusterProfiler"))
Each Bioconductor package contains at least one vignette, a document that provides a task-oriented description of package functionality. Vignettes contain executable examples and are intended to be used interactively.
You can access the PDF version of a vignette for any installed package from inside R using the browseVignettes()
function.
To view documentation about the DESeq2
package you can use the following R function:
browseVignettes("DESeq2")
1.3 Loading R and Bioconductor packages
To load an R package that has been installed on your local machine use the library()
function. For example, to load the ggplot2
package run:
library(ggplot2)
1.4 Learning resources
There are a multitude of learning resources for the R programming language. Here are just a few noteworthy examples that are entirely open source!!!